One of the major benefits of PHP is that it is independent platform, meaning it can be use on Mac OS, Windows, Linux and supports most web browsers. It also supports all the major web servers, making it easy to deploy on different systems and platforms at minimal additional cost.
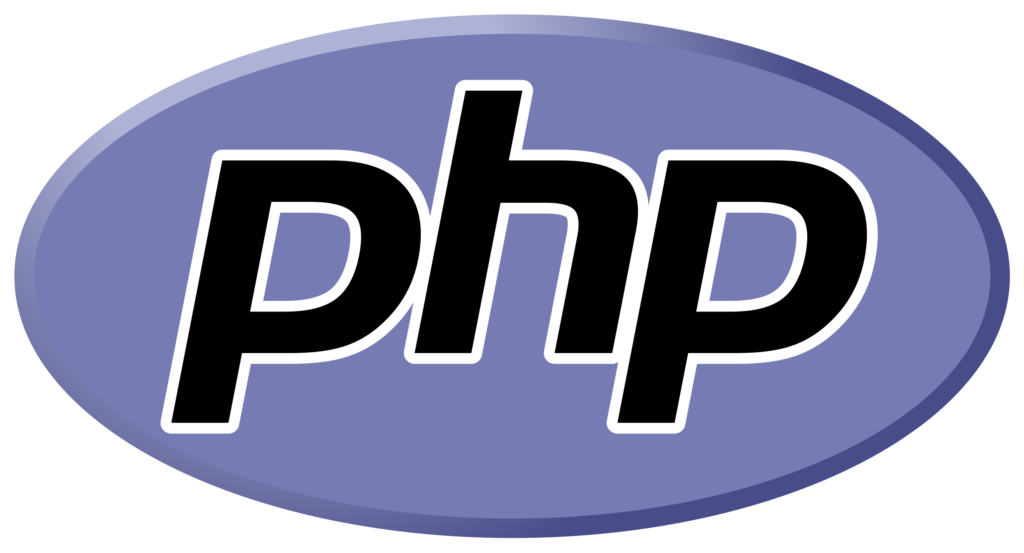
Why It is the future?
Since 75 percent of the web is powered by PHP there will obviously be a lot of jobs involving some sort of PHP coding. All these websites need to be maintained and there are PHP developers needed for that. The enormous market share of PHP won’t be gone overnight, so jobs involving PHP will be around in the future
CREATE YOUR fIRST php apP:
PHP is an incredibly powerful programming language, one that powers roughly 80% of the web! But it’s also one of the easier languages to learn as you can see your changes in real time, without having to compile or wait for the code to repackage your app or website.
Defining a PHP script:
To get started, create a file called “myfirstprogram.php.” You can actually call it anything you’d like, but the important part here is the extension: .php
. This tells the server to treat this page as a PHP script.
Now let’s go ahead….
create a basic HTML page:
<html>
<head>
<title>Hello</title>
</head>
<body>
Hello
</body>
</html>
save your page and upload it to any host that supports PHP. Now visit your page and you should see a page that give the outputs “Hello.”
Echo content:
Add some PHP code to our script. To signal the server to render PHP code we first open with the <?php
tag, then we write our PHP code, and finally close it with the ?>
tag.
write some PHP code that tells the server to echo specific output. To echo or print the content on the page we can use the echo
statement in our PHP code by placing the text we want to echo in single quotes and then end the command with a semi colon. Let’s echo out “there!”:
<html>
<head>
<title>Hello</title>
</head>
<body>
Hello <?php echo ‘there!’; ?>
</body>
</html>
Now Save your script and test it on your webhost. You should now see “Hello there!” on your screen.
echo Statement:
The echo
statement can be used with or without parentheses: echo
or echo()
.
<?php
echo “<h2>PHP is Fun!</h2>”;
echo “Hello world!<br>”;
echo “I’m about to learn PHP!<br>”;
echo “This “, “string “, “was “, “made “, “with multiple parameters.”;
?>
print Statement:
The print
statement can be used with or without parentheses: print
or print()
.
<?php
print “<h2>PHP is Fun!</h2>”;
print “Hello world!<br>”;
print “I’m about to learn PHP!”;
?>
Comments:
A comment in PHP code is a line that is not executed as a part of the program. Its only purpose is to be read by someone who is looking at the code.
Comments can be used to:
- Let others understand your code
- Remind yourself of what you did – Most programmers have experienced coming back to their own work a year or two later and having to re-figure out what they did. Comments can remind you of what you were thinking when you wrote the code
The syntax for single-line comments:
<!DOCTYPE html>
<html>
<body>
<?php
// This is a single-line comment
# This is also a single-line comment
?>
</body>
</html>
The syntax for multiple-line comments:
<!DOCTYPE html>
<html>
<body>
<?php
/*
This is a multiple-lines comment block
that spans over multiple
lines
*/
?>
</body>
</html>
Using comments to leave out parts of the code:
<!DOCTYPE html>
<html>
<body>
<?php
// You can also use comments to leave out parts of a code line
$x = 5 /* + 15 */ + 5;
echo $x;
?>
</body>
</html>